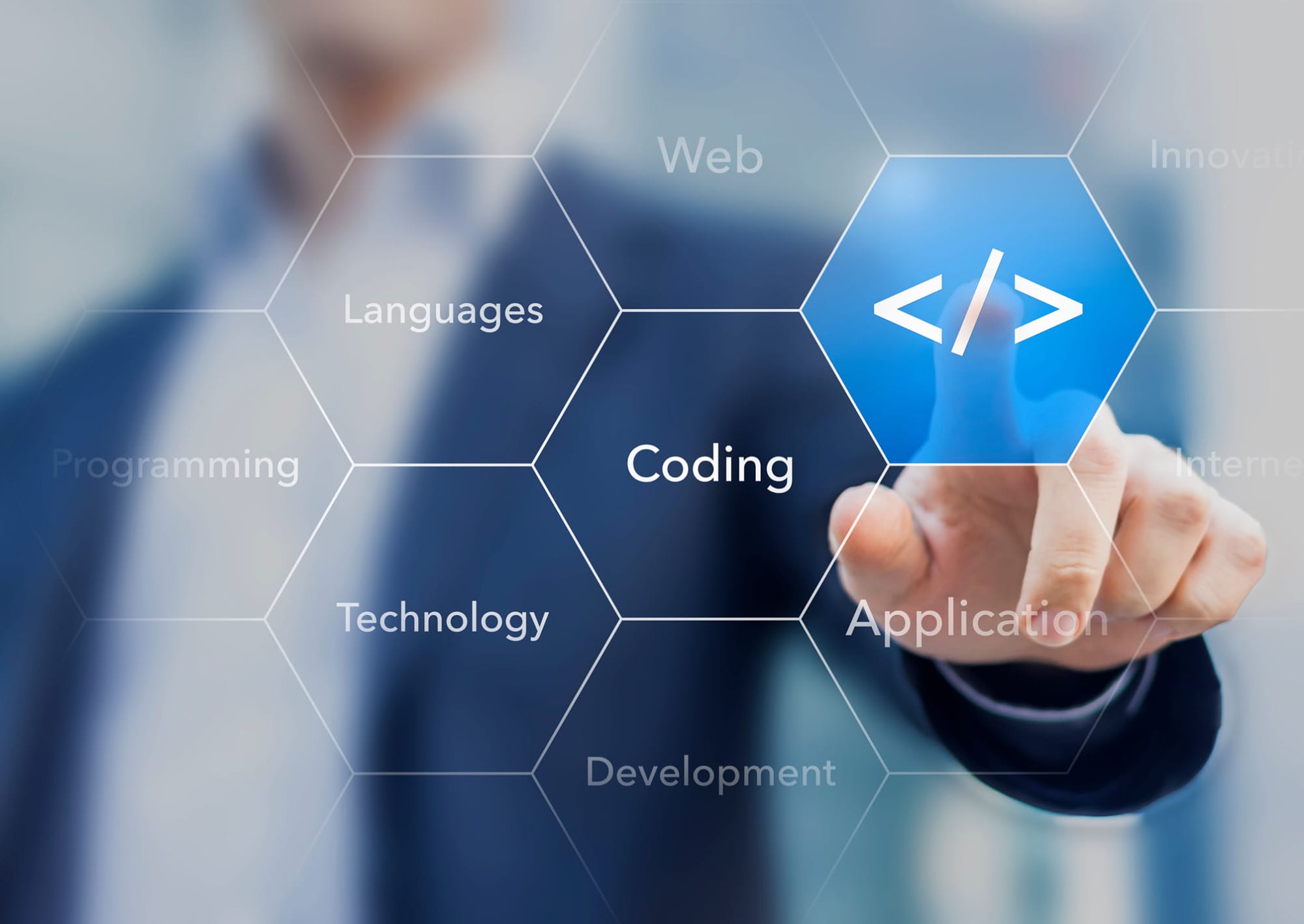
Course Duration
30 hrs
Medium of Instruction:
Live online sessions
Course Objective
The course helps acquire a fundamental understanding of the OOPs concepts, input/output data management, arrays in C++, functions, classes, objects, pointers, and much more. The course has been designed with a uniform structured series of modules enumerating various pertinent concepts.
Class Outcome
Upon completion of this course, students will be able to:
- Write C programs that are non-trivial.
- Use the variety of data types appropriate to specific programming problems.
- Utilize the modular features of the language.
- Demonstrate efficiency and readability.
- Demonstrate the use of the various control flow constructs.
- Use arrays as part of the software solution.
- Utilize pointers to efficiently solve problems.
- Include the structure data type as part of the solution.
- Create their own data types.
- Use functions from the portable C library.
Software Tools Used
Course Materials
Each student in our classes receives a comprehensive set of materials, including course notes.
C++ Language
Course Outline
Chapter 1: Setting up the tools
- Tools
- Installing C++ Compilers on Windows
- Installing VS Code on Windows
- Configuring Visual Studio Code for C++ on Windows
- Installing C++ Compilers on Linux
- Installing Visual Studio Code on Linux
- Configuring Visual Studio Code for C++ on Linux
- Installing C++ Compilers on MacOs
- Installing Visual Studio Code on MacOs
- Configuring Visual Studio Code for C++ on MacOs
- Online Compilers
Chapter 2: Diving in
- Your First C++ Program
- Comments
- Errors and Warnings
- Statements and Functions
- Data input and output
- C++ Program Execution Model
- C++ core language Vs Standard library Vs STL
Chapter 3: Variables and data types
- Variables and data types Introduction
- Number Systems
- Integer types : Decimals and Integers
- Integer Modifiers
- Fractional Numbers
- Booleans
- Characters And Text
- Auto
- Assignments
- Variables and data types summary
Chapter 4: Operations on Data
- Introduction on Data operations
- Basic Operations
- Precedence and Associativity
- Prefix/Postfix Increment & Decrement
- Compound Assignment Operators
- Relational Operators
- Logical Operators
- Output formatting
- Numeric Limits
- Math Functions
- Weird Integral Types
- Data Operations Summary
Chapter 5: Flow Control
- Flow Control Introduction
- If Statements
- Else If
- Switch
- Ternary Operators
- Flow Control Summary
Chapter 6: Loops
- Loops Introduction
- For Loop
- While Loop
- Do While Loop
Chapter 7: Object oriented Programming Concepts(Basic)
- Class
- Object
- Functions or Methods
- Inheritance
- Polymorphism
- Encapsulation
- Data hiding
Chapter 8: Advanced Topics